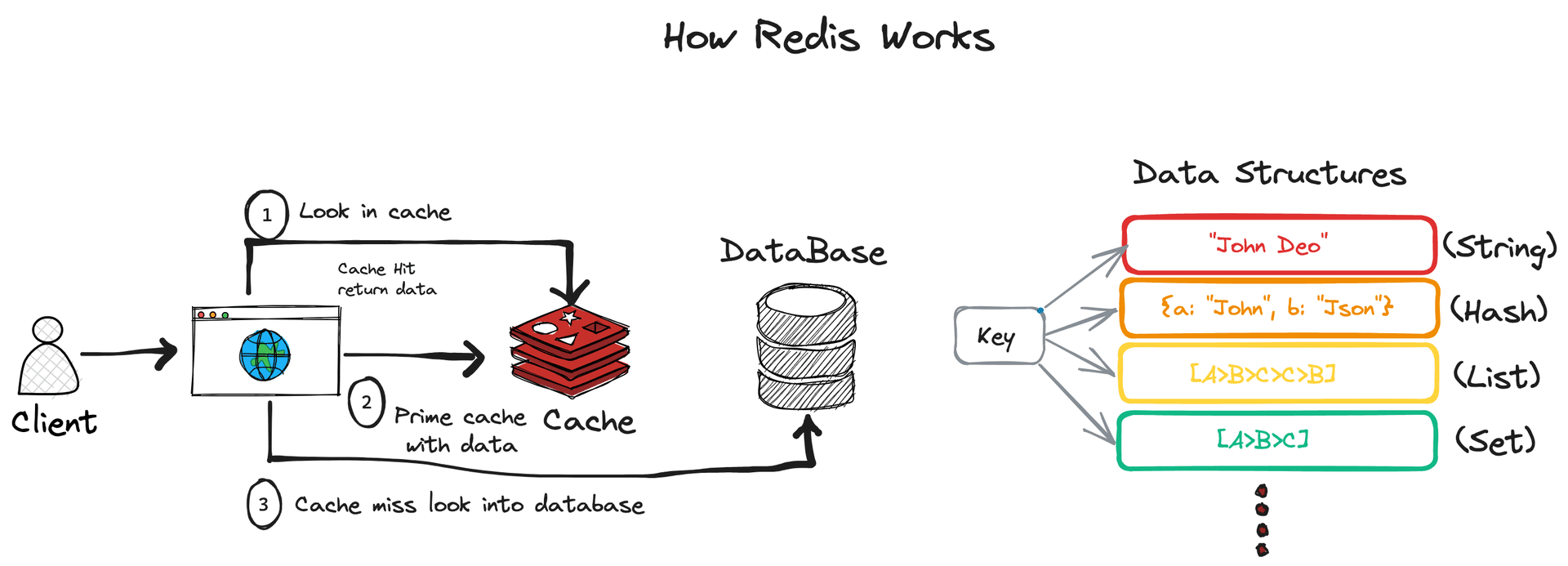
In today's world of fast-paced applications and data processing, efficient storage and retrieval mechanisms are crucial. Redis, an open-source, in-memory data structure store, has emerged as a powerful solution to address these challenges. This article explores various features and concepts of Redis through practical code examples in JavaScript.
Getting Started
Redis can be easily integrated into your applications using libraries like "ioredis." This library provides a user-friendly interface to interact with Redis servers. Let's dive into some essential Redis functionalities using the provided code snippets.
Connecting to Redis
To connect to a Redis server, we need to create a new instance of the Redis client. The client can be configured with the host and port of the Redis server. The default port is 6379.
import Redis from "ioredis";
const redisClient = new Redis({
port: 6379,
host: "127.0.0.1",
});
The code establishes a connection to a Redis server running locally on the default port (6379). The redisClient object allows us to interact with the server.
Basic Operations
Redis supports various data structures like strings, lists, sets, hashes, and sorted sets. Let's explore some of the basic operations on these data structures.
Strings
Strings are the simplest data structure in Redis. They can be used to store any type of data, including text, JSON, or binary data. Let's see how we can set and get a string value.
await redisClient.set("name", "John Doe");
const name = await redisClient.get("name");
console.log(name); // John Doe
Lists
Lists are ordered collections of strings. They can be used to store a sequence of data. Let's see how we can add and retrieve items from a list.
await redisClient.rpush("names", "John Doe");
await redisClient.rpush("names", "Jane Doe");
const names = await redisClient.lrange("names", 0, -1);
console.log(names); // ["John Doe", "Jane Doe"]
rpush
is used to add items to the end of a list.
lrange
is used to retrieve a range of items from a list. The first argument is the name of the list, and the second and third arguments are the start and end indices of the range. A negative index indicates the position from the end of the list.
Sets
Redis supports not only lists but also sets, which are unordered collections of unique elements.
const TAGS_SET_KEY = "tags";
await redisClient.sadd(TAGS_SET_KEY, "programming", "health", "business", "mindset");
const allTags = await redisClient.smembers(TAGS_SET_KEY);
console.log(allTags); // Output: ["programming", "health", "business", "mindset"]
await redisClient.srem(TAGS_SET_KEY, "business");
const remainingTags = await redisClient.smembers(TAGS_SET_KEY);
console.log(remainingTags); // Output: ["programming", "health", "mindset"]
sadd
is used to add items to a set.
smembers
is used to retrieve all the items in a set.
srem
is used to remove items from a set.
Hashes
Hashes are maps between string fields and string values. They are useful for storing objects. Let's see how we can store and retrieve an object using hashes.
const USER_HASH_KEY = "user:1";
await redisClient.hset(USER_HASH_KEY, "name", "John Doe");
await redisClient.hset(USER_HASH_KEY, "age", 30);
await redisClient.hset(USER_HASH_KEY, "email", "john@gmail.com");
const user = await redisClient.hgetall(USER_HASH_KEY);
console.log(user); // Output: { name: "John Doe", age: "30", email: "john@gmail" }
hset
is used to set a field in a hash.
hgetall
is used to retrieve all the fields and values in a hash.
Sorted Sets
Sorted sets are similar to sets, but each element is associated with a score. The elements are ordered by their scores. Let's see how we can add and retrieve items from a sorted set.
const USERS_SORTED_SET_KEY = "users";
await redisClient.zadd(USERS_SORTED_SET_KEY, 1, "John Doe");
await redisClient.zadd(USERS_SORTED_SET_KEY, 2, "Jane Doe");
await redisClient.zadd(USERS_SORTED_SET_KEY, 3, "Jack Doe");
const users = await redisClient.zrange(USERS_SORTED_SET_KEY, 0, -1);
console.log(users); // Output: ["John Doe", "Jane Doe", "Jack Doe"]
zadd
is used to add items to a sorted set. The first argument is the name of the sorted set, and the second argument is the score of the item.
zrange
is used to retrieve a range of items from a sorted set. The first argument is the name of the sorted set, and the second and third arguments are the start and end indices of the range. A negative index indicates the position from the end of the sorted set.
Expiring Keys
Redis allows us to set an expiration time for keys. Let's see how we can set an expiration time for a key.
await redisClient.set("name", "John Doe", "EX", 60);
The above code sets an expiration time of 60 seconds for the key "name." After 60 seconds, the key will be automatically deleted from the Redis server.
Working with Complex Data and Combining Data Types
Redis allows us to store complex data structures like objects and arrays. Let's see how we can store and retrieve an object.
const USER_HASH_KEY = "user:1";
const user = {
name: "John Doe",
age: 30,
email: "john@gmail.com",
interested: ["programming", "health", "mindset"],
};
await redisClient.hmset(USER_HASH_KEY, user);
const storedUser = await redisClient.hgetall(USER_HASH_KEY);
console.log(storedUser); // Output: { name: "John Doe", age: "30", email: "john@gmail", interested: ["programming", "health", "mindset"] }
const userInterested = await redisClient.hget(USER_HASH_KEY, "interested");
console.log(userInterested); // Output: ["programming", "health", "mindset"]
const userName = await redisClient.hget(USER_HASH_KEY, "name");
console.log(userName); // Output: John Doe
hmset
is used to set multiple fields in a hash.
hgetall
is used to retrieve all the fields and values in a hash.
hget
is used to retrieve a specific field from a hash.
Transactions
Redis allows us to execute multiple commands as a single atomic operation. Let's see how we can use transactions.
await redis.multi()
To run transactions, we can start by using the multi
function. This by default, will automatically create a pipeline as well.
const results = await redis.multi()
.hset("user-purchase","customer", "keith")
.hset("user-purchase", "amount", 100)
.incr("customer-keith-purchase-count")
.exec()
console.log(results) // [ [ null, 0 ], [ null, 0 ], [ null, 9 ] ]
Finally, to send all commands, we can use the exec
function on the pipeline. Redis will execute all the commands and returns the results in an array.
if you would like to disable transactions, you can set the named parameter pipeline=False
.
await redis.multi({ pipeline: false })
Pipelines
Redis allows us to send multiple commands to the server without waiting for the replies at all, and finally read the replies in a single step. This is called a pipeline. Let's see how we can use pipelines.
const pipeline = redis.pipeline()
To run pipelines, we can start by using the pipeline
function. This by default, will automatically create a pipeline as well.
const results = await redis.pipeline()
.hset("user-purchase","customer", "keith")
.hset("user-purchase", "amount", 100)
.incr("customer-keith-purchase-count")
.exec()
console.log(results) // [ [ null, 0 ], [ null, 0 ], [ null, 9 ] ]
Finally, to send all commands, we can use the exec
function on the pipeline. Redis will execute all the commands and returns the results in an array.
Conclusion
Redis is a dynamic and efficient data store offering a wide array of features to accommodate diverse use cases. In this article, we've explored basic key-value storage, list and set operations, pipelines for efficient command execution, and complex data storage. By utilizing the "ioredis" library and JavaScript, developers can harness Redis's power to optimize their data management strategies.
Always remember to disconnect from the Redis server using redisClient.quit() once you've completed your operations, ensuring proper resource management.
These code examples provide a foundation for leveraging Redis effectively. Whether you're building real-time applications, caching systems, or managing distributed environments, Redis can significantly enhance your application's performance and scalability, providing a reliable and versatile solution for modern software development.